In PowerShell, foreach is a keyword that allows you to execute a set of statements against every item in a collection or array. Now that we have the editor-pleasing succinct definition to start this off, we can dive into what that means. First, by breaking down all the options of foreach. Who doesn’t love a good pro/con list to help them pick the right option?
Foreach options
Foreach-Object is a cmdlet. In most cases, it is your slowest option because it processes each entry one at a time. Part of what makes it so confusing is it has an alias of foreach, which is the same as the keyword. A good way to know which you are using is to look for a pipe. Only the cmdlet is going to allow data in through the pipe. While slower, it comes with the ability to pass the results into another command.
Foreach keyword loads all items into memory and then runs. This is usually significantly faster as long as you have the required memory to run it. It is my usual go-to; I love the speed, and I rarely deal with data sets so large my memory could become an issue.
Foreach method came around in PowerShell 4 and is surprisingly difficult to find information about. If you run Get-Member for a collection, it won’t even mention foreach as a method. What we do know is that it is the fastest option you have. If you are looking to kill a lot of processes on the fly or change data types for your collection, this option will crush it.
Foreach for data cleanup
It is uncommon for data you have collected to be exactly what you wanted, whether you need to convert bits of information to something new or remove all items from your collection that don’t meet the parameters you are looking for. Let's say you are given a CSV file of action heroes; if we read that list, it looks like this:
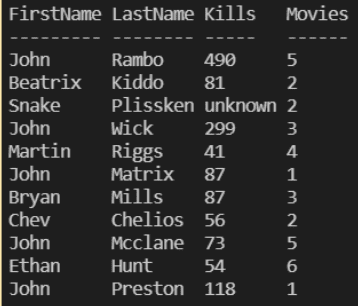
That is an awesome list, and whoever had this idea is probably pretty cool, but what if we don’t need everything from it? This is where we can use foreach. In this first example, we will trim this list down to only include action heroes named John.
$ActionHero = Import-CSV "C:\Temp\john.csv"
$FinalList = New-Object 'System.Collections.ArrayList'
foreach($Hero in $ActionHero){
If($Hero.FirstName -eq "John"){
$FinalList.Add($Hero) > $null
}
}
This option takes each hero named John and builds a new collection that looks like this.
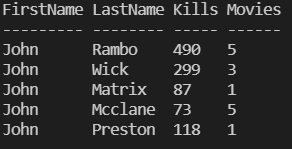
We can take that array and build a new CSV with only the data we want. Having PowerShell there to clean up old or junk data and whittling it down to what is relevant is very valuable for creating colorful charts and graphs that management can ooh and aah over, making you look like a rockstar.
Foreach for running commands
More than just cleaning up data, foreach can also be used to run a series of commands against many items in short order. Let’s say I have been fired because I made one too many snide remarks against management in my blogs. I have been right about management this entire time, so instead of service accounts or LAPS, we have a local admin account that has the same name and password on each server. Who knows what evil deeds I could do if I so desired. With foreach, we can grab every computer and reset the password.
$computers = Get-ADComputer -filter * | Select-Object DNSHostname
$cred = Get-Credential
foreach($computer in $computers){
$LocalAdmin = [ADSI] "WinNT://$computer/LocalAdminGuy"
$LocalAdmin.SetPassword($cred.GetNetworkCredential().password)
}
Now, every machine has a new local admin. While we are concerned about this, I would maybe suggest disabling that account and implementing LAPS…or I would if I had not been let go mid-blog!
Conclusion
Foreach is very powerful; it allows you to take large collections and either sift through the data or make large-scale changes very quickly and easily. Now go forth and collect all the data your executive has been telling you they needed last week…so they can ignore it for a month and ask for it again when they forget you already sent it. It is the corporate way!