You know those fancy popups that appear in the bottom corner of your computer screen? Those are called toast notifications. Have you ever wondered if there’s a way to create your own customized toast notification? Perhaps you wrote a script that you want a notification for upon completion. Do your users forget to reboot their machines, and you want to send them a reminder? Fret not, my friend — there is hope.
BurntToast PowerShell module
Luckily for us, there’s a PowerShell module for displaying toast notifications: BurntToast. There’s something almost magical about being able to easily display toast notifications just by running a line of PowerShell. I’m a fan of anything that extends the PowerShell experience outside of the console. Let’s get started by installing the module.
Install-Module -Name BurntToast
Using the main command from the BurntToast module, New-BurntToastNotification, create a test toast.
New-BurntToastNotification -Text 'Yoooo, this is a toast notification... from PowerShell!'
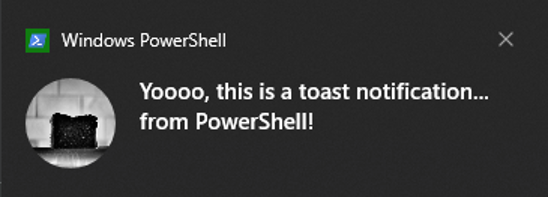
This is a relatively simple example, but toasts can be customized as needed. You can place a button on toasts that can take you to places all over the web:
$BlogButton = New-BTButton -Content "Read Blog" -Arguments 'https://pdq.com/blog'
New-BurntToastNotification -Text 'Amazing content awaits you' -Button $BlogButton
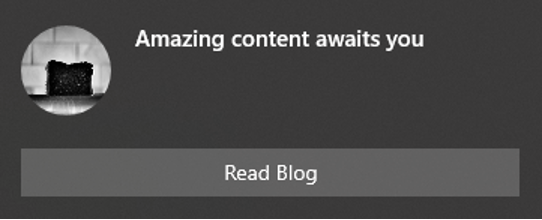
You can even customize the sound or add a Snooze/Dismiss button.
New-BurntToastNotification -Text 'Cookies are done' -SnoozeAndDismiss -Sound SMS
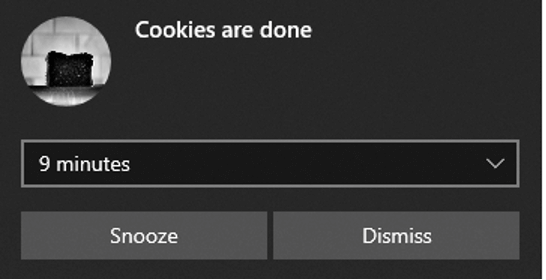
BurntToast Pomodoro Timer
If you are anything like me, staying on task can be a challenge. I’ve found that working in 25-minute increments followed by a five-minute break helps me get more done and ensures that I take adequate breaks. Turns out this approach is called the Pomodoro Technique. Let’s create a Pomodoro Timer with PowerShell that displays a toast notification upon completion.
This function needs to do two things: wait for 25 minutes and then display a toast. Just running Start-Sleep would cause the PowerShell console to be busy, so we can leverage PowerShell background jobs so that we can still use our PowerShell Window during the Pomodoro timer.
Define the function in the active PowerShell console.
Function Start-PomodoroTimer {
Param(
$Minutes = 25
$seconds = 60 * $Minutes
$sb = {
Start-Sleep -Seconds $using:seconds
New-BurntToastNotification -Text 'Timer complete. Take a break and get back to it' -SnoozeandDismiss -Sound SMS
}
Start-Job -Name 'Pomodoro Timer' -ScriptBlock $sb -Argumentlist $seconds
}
Once the function is defined, simply run Start-PomodoroTimer and get to work! This will start a job in the background that waits a specified number of minutes and then displays a toast.
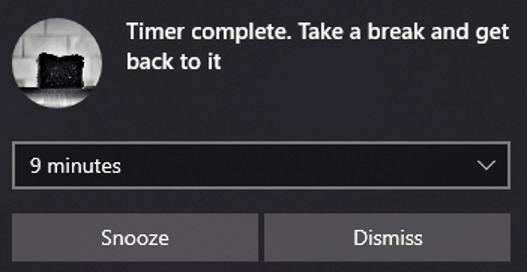
I save this function to my PowerShell profile.ps1 file and regularly use it.
Looking for other PowerShell pro tips?
Here are three of our favorite PowerShell modules.
Deploying a toast notification
Do you have any users who never reboot their machine? You can automate away your reboot reminders using PDQ Deploy and Inventory, PowerShell, and BurntToast. Let’s create a package in PDQ Deploy that will show a toast notification to users that need to reboot their machines for one reason or another. The idea and process behind this notification can be applied to any other time you’d need to display a notification on a machine.
Let’s create a PowerShell script that we can deploy to computers.
The first thing we need to tackle is installing the BurntToast module on our targets. We can do this by modifying the Install and Import Module script from the PowerShell-Scanners repository: PowerShell-Scanners/Install and Import Module.ps1 at master · pdq/PowerShell-Scanners (github.com)
$ModuleName = 'BurntToast'
# Install the module if it is not already installed, then load it.
Try {
$null = Get-InstalledModule $ModuleName -ErrorAction Stop
} Catch {
if ( -not ( Get-PackageProvider -ListAvailable | Where-Object Name -eq "Nuget" ) ) {
$null = Install-PackageProvider "Nuget" -Force
}
$null = Install-Module $ModuleName -Force
}
$null = Import-Module $ModuleName -Force
This will ensure that the module is downloaded on the computer that it is being run on. Next, we need to create the actual toast notification. For this one, we will keep it simple and elegant.
New-BurntToastNotification -Text "This computer hasn't been rebooted in $uptimeDays days. Please reboot when possible." -SnoozeAndDismiss
To add a bit of customization to our script, we are going to check the uptime on the computer. If it’s less than seven days, we will exit out of the script with a return code of 0.
$Days = 7
# Check the uptime
$cim = Get-CimInstance win32_operatingsystem
$uptime = (Get-Date) - ($cim.LastBootUpTime)
$uptimeDays = $Uptime.Days
# return code 0 if this computer hasn't been online for too long
if ($uptimeDays -LT $Days) {
Exit 0
}
Here is the completed script, to be saved as a .ps1 file. Make sure to always test, modify (if needed), and verify any code before running it in your environment. This script has a Days parameter that can be used to specify the minimum number of days for a computer to be online before displaying a message. If the computer hasn’t been online longer than the Days parameter, the script will exit and not display a toast.
[CmdletBinding()]
param(
$Days = 7
)
$ModuleName = 'BurntToast'
# Check the uptime
$cim = Get-CimInstance win32_operatingsystem
$uptime = (Get-Date) - ($cim.LastBootUpTime)
$uptimeDays = $Uptime.Days
# return code 0 if this computer hasn't been online for too long
if ($uptimeDays -LT $Days) {
Write-Verbose "Uptime is $uptimeDays days. Script will not proceed" -Verbose
Exit 0
}
# Install the module if it is not already installed, then load it.
Try {
$null = Get-InstalledModule $ModuleName -ErrorAction Stop
} Catch {
if ( -not ( Get-PackageProvider -ListAvailable | Where-Object Name -eq "Nuget" ) ) {
$null = Install-PackageProvider "Nuget" -Force
}
$null = Install-Module $ModuleName -Force
}
$null = Import-Module $ModuleName -Force
Write-Verbose "Displaying Toast" -Verbose
New-BurntToastNotification -Text "This computer hasn't been reboot in $uptimeDays days. Please reboot when possible." -SnoozeAndDismiss
Now, we need to package up this script and deploy it using PDQ Deploy. Make sure to save your script as a .ps1 file and put it into a location that works for your environment, whether that be a network share or your PDQ Deploy Repository. Create a new package in PDQ Deploy and set the conditions so that it only runs on supported operating systems. In my testing, this worked on Windows 10, Windows 11, Server 2019, and Server 2022. Set the Logged On State condition so that it only runs if someone is logged on.
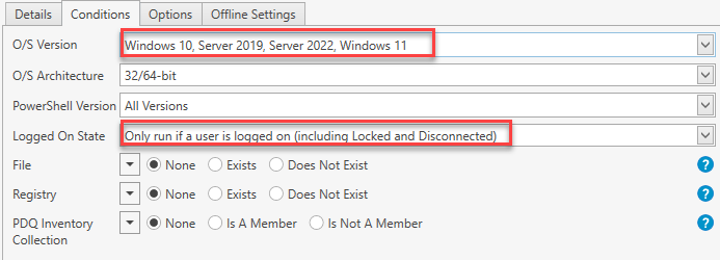
Toast notifications need to be run in the context of the logged-on user. On the options tab, set the Run As mode accordingly.
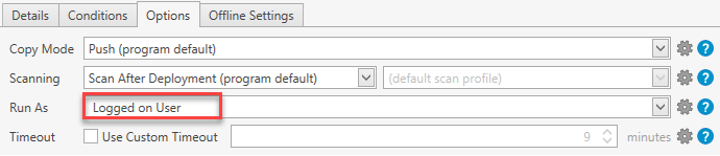
Next, add an Install step and select the script that you recently saved.
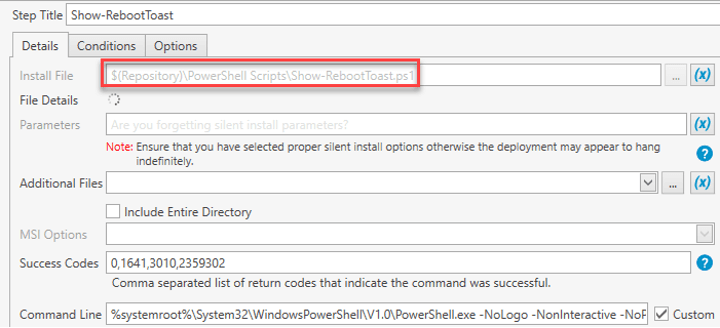
You can use the Parameters field to choose a different number of days, and setting it to –Days 0 will show it for all computers.
If the computer that you deploy this to hasn’t been rebooted in seven days, the logged-on user should see something like this after deploying the package to them:
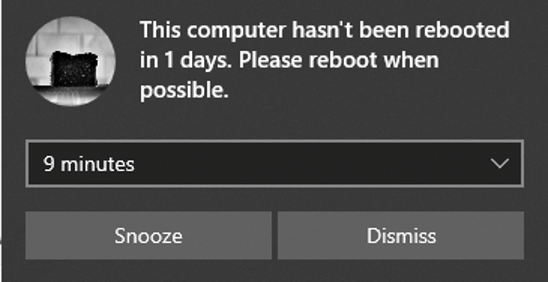
BurntToast is a great tool to add to your toolbelt, and I regularly find reasons to add it to my scripts. We only covered a small amount of what you can do with BurntToast. To learn more, check out the Github page. If you like PowerShell and want to hear more, check out the PowerShell Podcast.
Loading...