In PowerShell, an if
statement lets you run code only when a condition is true. It’s one of the most fundamental tools in any programming language, and in PowerShell, it’s essential for writing scripts that respond to different situations or outcomes.
The TL;DR
PowerShell if statements let you run code only when a condition is true.
if (condition) { code }: Basic structure
Add else / elseif for more options
Use -and, -or, -not to combine or negate conditions
If statements work great for checking files, values, or system states
Example: if ($day -eq 'Tuesday') { "Taco Tuesday" }
Smart scripts start with smart logic.
Conditional logic gives your script the ability to make decisions — if something is true, run this; if not, do something else. Whether you're checking a file path, comparing numbers, or branching workflows, if
statements help automate your logic in a smart, readable way.
Still a bit abstract? Think of it like this:
If I’m hungry, I eat.
If I’m tired, I nap.
If my PowerShell script finds a certain condition to be true... it acts.
If a condition is true, it runs the code. If it’s not, it skips it.
To see this in action, we’ll start with a quick diagram and then look at a working PowerShell example.
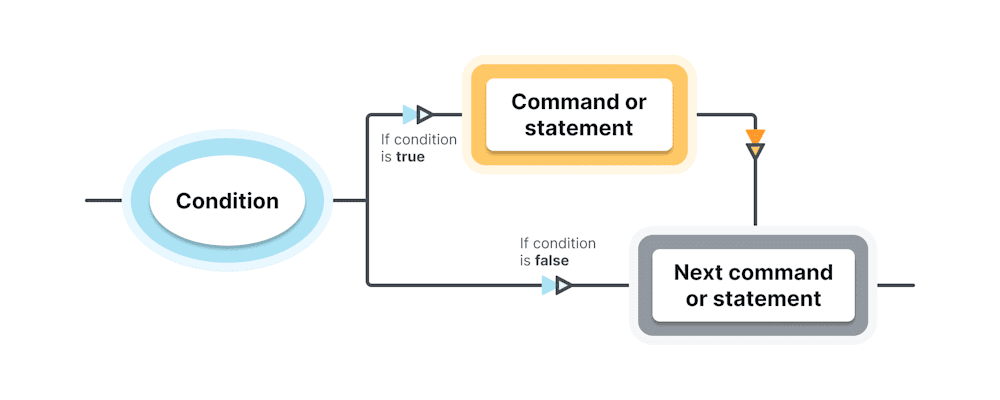
The fundamentals of PowerShell if statements
When a PowerShell script encounters an if
statement, it evaluates the condition inside the parentheses. If that condition is true
, PowerShell runs the block of code inside the curly braces. If it’s false
, PowerShell skips over that block and continues to the next step.
Example: A basic PowerShell if statement
Let's say you're keeping track of how many eggs you have:
$eggs = 10
if ($eggs -lt 12) {
"You have less than a dozen eggs."
}
In this example,
The variable
$eggs
is set to10.
The condition
($eggs -lt 12)
checks whether that number is less than12.
Since the condition is
true
, PowerShell runs the code inside theif
block and returns:"You have less than a dozen eggs."
Now, if you change the value of $eggs
to 13
, the condition becomes false
, and nothing happens. PowerShell skips the message and moves on.
This is how if
statements control the flow of your script — they act only when a condition is met.
That’s the basics. Now let’s peel back the curtain and look at how if
statements handle more complex decisions. Because your script, much like your day, probably has more than one thing to deal with. Let’s break down the syntax behind it and explore how to handle multiple conditions.
PowerShell if statement syntax
The syntax of an if
statement in PowerShell is straightforward and resembles other coding languages.
if (condition)
{statement or command}
The condition goes inside parentheses.
The code to run if the condition is true goes inside the curly braces.
If the condition is false, the code block is ignored.
Common comparison operators
Most if
statements use comparison operators to evaluate values. These are some of the most common in PowerShell:
Using these operators gives your conditions structure and power, because you want your scripts to behave how you'd want, right?
Using cmdlets inside if statements
You’re not limited to comparison operators. You can also use cmdlets directly inside if
statements. For example:
if (Test-Path 'c:\temp\macgyver_biography.txt') {
Get-Content 'c:\temp\macgyver_biography.txt' | Measure-Object -Word
}
And a visual representation:
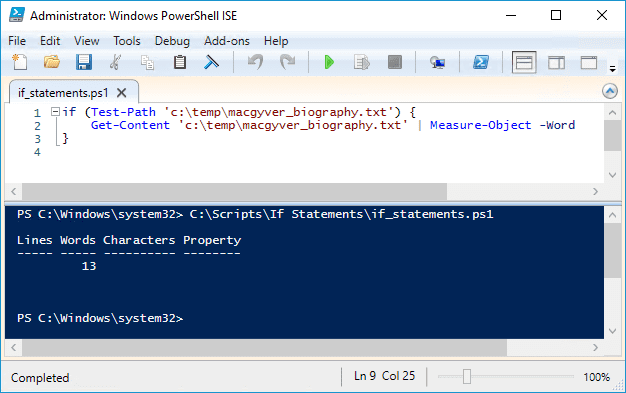
Here's what's happening:
Test-Path
checks whether a file exists at the given pathIf it does, the condition is true, and the script runs the block
The block reads the file and counts the number of words using
Measure-Object
If the file does not exist, then the condition evaluates to false and the script just ends.
As you can see from the screenshot, my MacGyver biography is only 13 words long so far. One of these days I'll finish it.
Easily run PowerShell scripts on remote devices
Need to run your awesome PowerShell scripts on remote devices? PDQ Connect can easily execute PowerShell scripts on any managed device with an active internet connection.
PowerShell if-else statements
Up to this point, we've seen how if
statements run code when a condition is true. But what if the condition isn't true? That's where the else
statement comes in.
An if-else
structure lets you define two possible outcomes: one if the condition is true, and another if it's false. It’s a simple way to ensure your script always has a response, even when the condition doesn’t go your way.
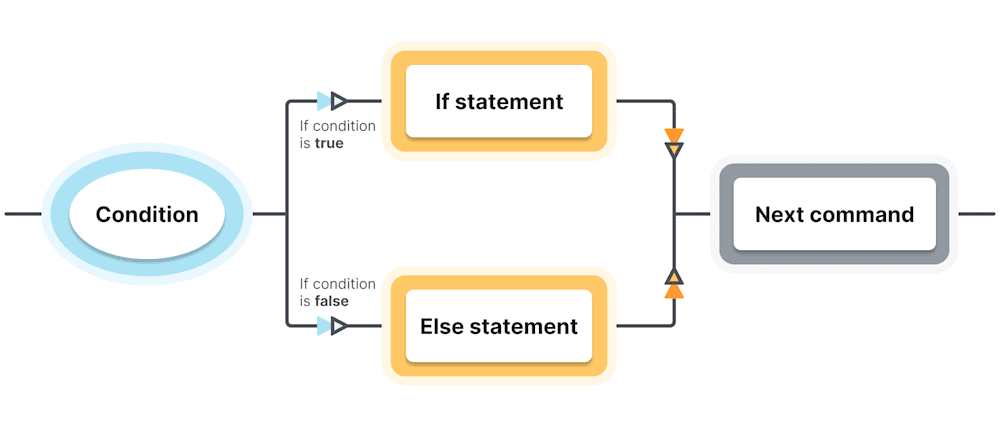
In this diagram, you can see that we now have two statements that can be executed: one statement if the condition returns true, and one statement if the condition returns false.
Example: Basic PowerShell if statement syntax
$x = 4
if ($x -ge 3) {
"$x is greater than or equal to 3"
}
else {
"$x is less than 3"
}
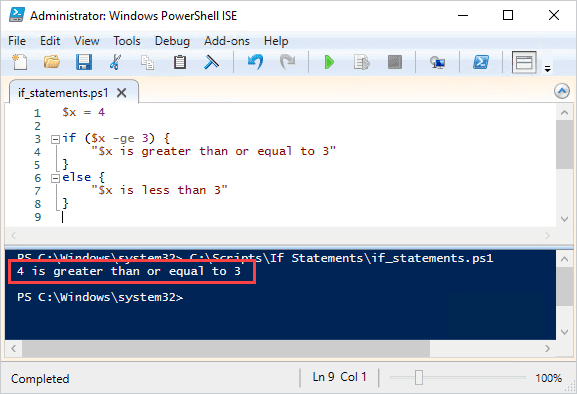
Here's how this example breaks down:
The variable
$x
is set to4
The condition
($x -ge 3)
checks whether$x
is greater than or equal to 3Since the condition is true, PowerShell runs the
if
block and returns:"4 is greater than or equal to 3"
Let's flip the condition
Change the value of $x
to 1
and run the same logic:
$x = 1
if ($x -ge 3) {
"$x is greater than or equal to 3"
}
else {
"$x is less than 3"
}
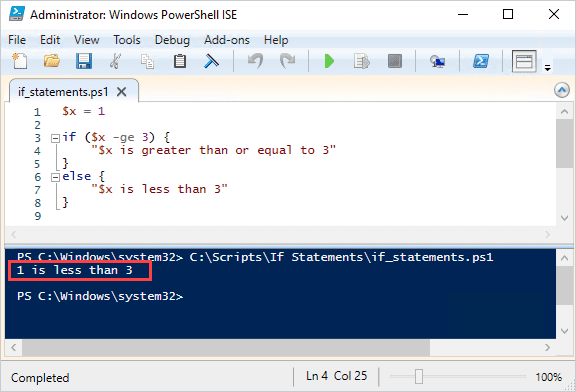
This time, the condition is false, so PowerShell skips the if
block and executes the else
block instead, returning
"1 is less than 3"
By using else
, you're giving your script a fallback plan, or rather something to do when the first condition doesn’t match. It's an easy way to cover both sides of a decision without writing multiple separate checks.
Nested if statements in PowerShell
Sometimes your script needs to make a decision after making another decision. That’s where nested if
statements come in. Instead of evaluating everything at once, you evaluate one condition first, then, inside that block, you check something else.
In other words, if this is true, then check this other thing.
Example: Check if a folder and file exist
$directoryPath = "C:\Files"
$file = "PC_Info.csv"
$fullPath = Join-Path $directoryPath $file
if (Test-Path -Path $directoryPath){
if (Test-Path -Path $fullPath){
"File exists"
}
else {
"File doesn't exist"
}
}
else {
"Directory doesn't exist"
}
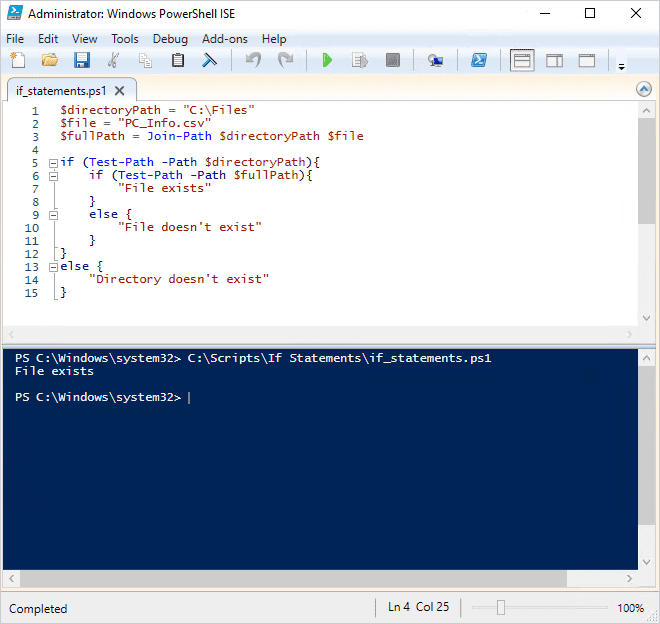
This example script checks to see if a directory exists. If the directory exists, it checks to see if a particular file exists.
Breaking this example down:
You define a folder path and a file name, then combine them into a full path
PowerShell checks if the folder exists with
Test-Path
If the folder doesn’t exist, it skips everything else and returns:
"Directory doesn't exist"
If the folder does exist, PowerShell continues and checks if the file exists
If the file exists:
"File exists"
If it doesn’t:
"File doesn't exist"
PowerShell elseif statements
Using elseif
allows your script to evaluate multiple conditions in sequence. PowerShell checks each one in order, and as soon as it finds a condition that’s true, it runs that block and stops checking the rest.
This makes elseif
perfect for clean, readable logic when you're comparing a single value against multiple outcomes.
Example: Evaluating how many eggs you have
Yes, I'm bringing back the eggs.
$eggs = 14
if ($eggs -eq 12) {
"You have exactly a dozen eggs."
}
elseif ($eggs -lt 12) {
"You have less than a dozen eggs."
}
else {
"You have more than a dozen eggs."
}
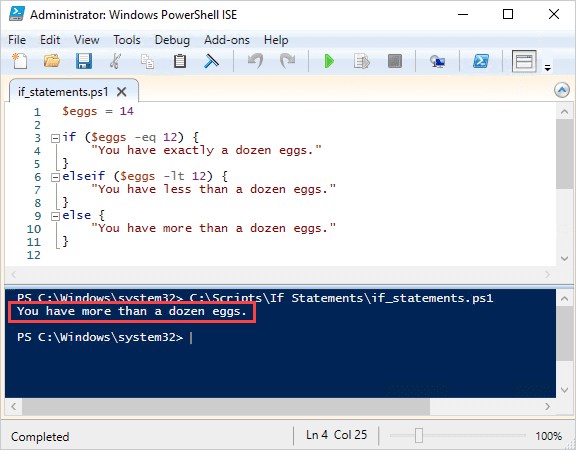
And how this works:
PowerShell starts by checking if
$eggs
is equal to 12If true, it runs that block and skips the rest
If not, it moves on to the
elseif
and checks if$eggs
is less than 12If neither condition is true, PowerShell hits the
else
block as a final fallback
This structure is often easier to follow than nesting multiple if
statements, especially when you're checking the same variable against different values. Think of elseif
as the more elegant alternative to over-nesting. (Did that chicken-nest pun land?)
Combining multiple condition expressions using -and and -or
PowerShell lets you combine multiple conditions inside a single if
statement using logical operators like -and
and -or
.
This is especially useful when you want to check for multiple scenarios at once instead of writing separate if
statements for each condition.
Use
-and
when all conditions must be trueUse
-or
when any one condition can be true
Example: Check if both Chrome and Firefox are running
if ((get-process chrome -ErrorAction SilentlyContinue) -and (get-process firefox -ErrorAction SilentlyContinue)){
"Chrome and Firefox are running"
}
else {
"Both Chrome and Firefox are not running"
}
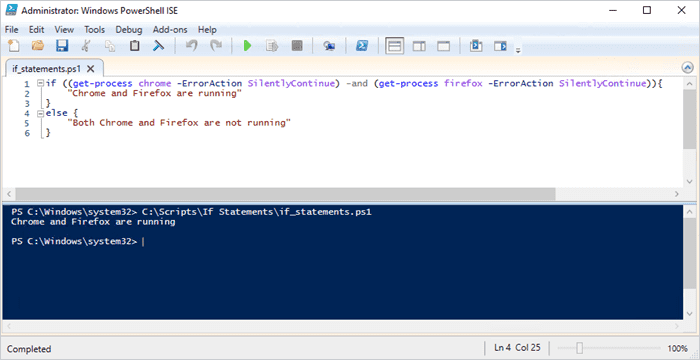
In the example above, both Chrome and Firefox must be running for the condition to return true.
What’s happening here:
PowerShell checks for both processes using
Get-Process
The
-ErrorAction SilentlyContinue
prevents errors if the processes aren’t foundIf both Chrome and Firefox are running, the
if
block is triggeredIf one or both are missing, the
else
block runs
Example: Check if Chrome or Firefox is running
If you want your condition statement to return true as long as one of the expressions returns true, use the -or operator.
if ((get-process chrome -ErrorAction SilentlyContinue) -or (get-process firefox -ErrorAction SilentlyContinue)){
"Chrome or Firefox are running"
}
else {
"Neither Chrome or Firefox are running"
}
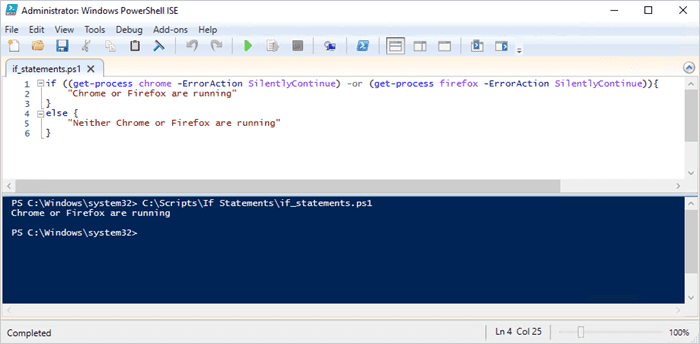
In this example, we used the -or operator. The condition returns true as long as Chrome or Firefox is currently running:
PowerShell still checks for both processes
But now, the
if
block is triggered if either one of them is runningOnly if neither is found does the
else
block execute
This kind of logic comes in handy when you’re building conditionals that depend on environment checks, multiple system states, or fallback scenarios.
How to negate PowerShell if statements
Sometimes with operators, we need to negate the statement.
In PowerShell, you can negate a condition using:
-not
(more readable)!
(shorter, more common in scripts)
Both do the same thing. They flip the result of a condition from true to false, or vice versa.
Example: Check that a file does not exist
Using the earlier MacGyver example, what if instead of searching for the MacGyver biography, we want to make sure it isn't there? Here's an example of how to do that:
if (!(Test-Path 'c:\temp\macgyver_biography.txt')) {“This Machine lacks the biography you need, perhaps you can create on with a paperclip and a matchstick.”}
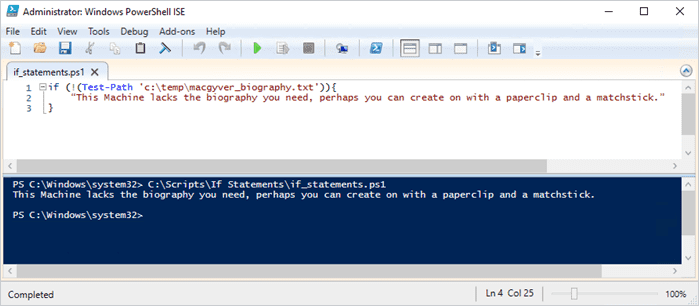
What's happening here:
Test-Path
checks whether the file existsThe
!
operator negates the resultSo, if the file does not exist, the condition evaluates to true, and the message runs
If the file does exist, the script skips the message
PowerShell dinner menu
It’s time to put all this PowerShell if
statement knowledge to good use. Let’s use several of the principles we covered in this article to create a script that gives us our dinner plans depending on what day of the week it is.
First, let’s get the day of the week using the Get-Date cmdlet, returning the DayOfWeek property and assigning it to the $day variable.
$day = (Get-Date).DayOfWeek
Next, we'll build our nested conditional statement for the different days of the week and assign a different meal for each day.
if ($day -eq 'Monday') {
"Macaroni Monday"
}
elseif ($day -eq 'Tuesday') {
"Taco Tuesday"
}
elseif ($day -eq 'Wednesday') {
"Waffle Wednesday"
}
elseif ($day -eq 'Thursday') {
"Tilapia Thursday"
}
elseif ($day -eq 'Friday') {
"Falafel Friday"
}
elseif ($day -eq 'Saturday') {
"Sushi Saturday"
}
else {
"Schnitzel Sunday"
}
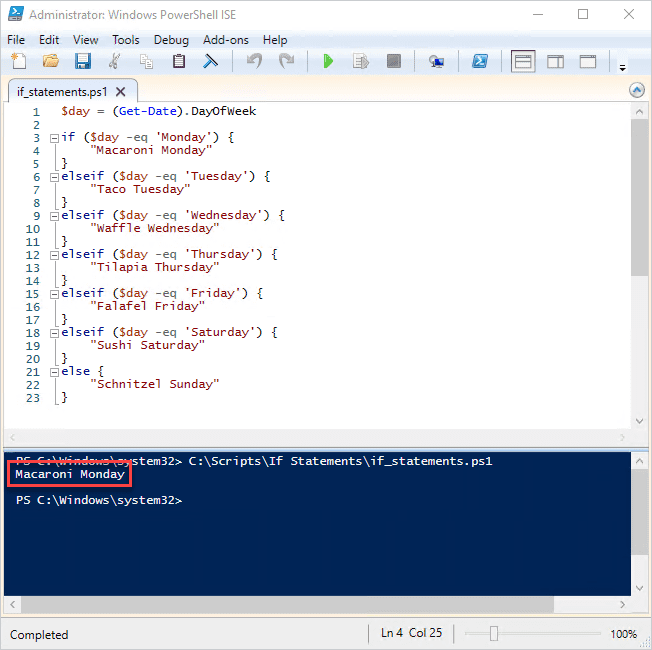
Since I ran this command on a Monday, the returned dinner plan was "Macaroni Monday."
While this script runs as planned and returns the correct results, I need to add a caveat. If you are using several elseif
statements in the same command, you may want to consider using a switch statement instead. Switch statements can accomplish the same thing as multiple elseif
statements, but are much easier to read, and readability is a big factor when making a PowerShell scripts. If only we had an article that covered PowerShell switch statements….
Wrapping up
PowerShell if
statements may be simple at their core, but as you've seen, they unlock a ton of flexibility in your scripts, from one-liner checks to full-blown decision trees. Whether you're tracking eggs, scanning for running processes, or building out a weekday dinner menu, conditional logic helps you write scripts that actually think.
Want to take this knowledge even further? PDQ Connect makes it easy to run PowerShell scripts on remote machines, which is perfect for when you need that if
block to evaluate across dozens or hundreds of endpoints. And if you want even more scripting insights, use cases, and slightly off-the-rails banter, check out The PowerShell Podcast, where we talk all things PowerShell, automation, and sysadmin life.
Your scripts are about to get a whole lot smarter. Happy scripting.