PowerShell scripts often require a specific file to exist for the script to function. When the file is not there, your script will throw up an error instead of just moving on, breaking the flow of your scripts and testing your PowerShell error handling skills. We could absolutely set that error to continue silently, but wouldn’t it be better to know if this critical file does, in fact, exist? Enter Test-Path.
Grabbing the file vs. Test-Path
To test this, let us use the probably-not-true scenario of me copying my Social Security number to several computers on a drunken dare. Upon sobering up, I want to correct this, but I have no idea which machines I put the information on. This is time sensitive, so I don’t want to go to each machine and test it individually, but I need to check every machine and remove the file if it exists. If I attempt to grab the file and am wrong about its existence, I will fail with the following error:

Test-Path is not trying to return with the object, so it just returns whether the file exists.

With no error, we do not have to worry about our script just stopping if the file does not exist. Instead, it opens a few possibilities.
Copy the file to the machine
One common option is to copy the file over to the machine if it does not exist. The exclamation point in the following script tells it to proceed if Test-Path returns a false value.
if(!(Test-Path -Path C:\Temp\JordansSocial.txt)){
Copy-Item "\\RealFileServer\CriticalFiles\JordansSocial.txt" -Destination "C:\temp\Jordanssocial.txt"
}
This script is of immense value if an install or custom software requires a certain file to work. It allows you to make sure everything you need for a script to succeed is present before continuing. In my situation, this is a worst-case scenario, as it checks a computer and copies over my private information if it wasn’t already there. This is just compounding my issue.
Delete a file with a known name
What I am looking for is to delete the item if it exists and give me a nice message if it does not.
$JordansShame = "C:\Temp\JordansSocial.txt"
if(Test-Path -Path $JordansShame){
Remove-Item $JordansShame
}Else{
Write-Host "It turns out your stupidity does know SOME bounds"
}
Problem solved … assuming I named the file the same thing. I can’t be sure I did, and we need to protect my super sweet 375 credit score. It is time to flex some of the versatility of Test-Path.
Check C:\temp
Let’s define some known values and see if we can’t define a scenario where I catch all my files.
We know I started drinking 11 hours ago.
I lack creativity and always copy files into C:\temp. I have six years of webcasts backing this up.
Knowing this, I need a script to look in C:\temp for any file that was created within the last 11 hours and remove it.
$date = (Get-Date).AddHours(-11)
$JordansShame = “C:\Temp\”
if(!(Test-Path -Path $JordansShame -NewerThan $date)){
Write-Host "No new files created within the last 11 hours"
}else{
Get-ChildItem $JordansShame | Where-Object{$_.LastWriteTime -gt $date} | Remove-Item
}
Combine this script with Invoke-Command, and we can clear out my mistake from every computer.
$computers = "Billy.whiskeytime.club","Demogorgon.whiskeytime.club","Dustin.whiskeytime.club","Eddie.whiskeytime.club","Eleven.whiskeytime.club","Hopper.whiskeytime.club"
$cred = Get-Credential
$script = {
$date = (Get-Date).AddHours(-11)
$JordansShame = "C:\temp\"
if(!(Test-Path -Path $JordansShame -NewerThan $date)){
Write-Host "No new files created within the last 11 hours on $(hostname)"
}else{
Write-Host "Deleting files from $(hostname)"
Get-ChildItem $JordansShame | Where-Object{$_.LastWriteTime -gt $date} | Remove-Item
}
}
Invoke-Command -ComputerName $computers -ScriptBlock $script -Credential $cred
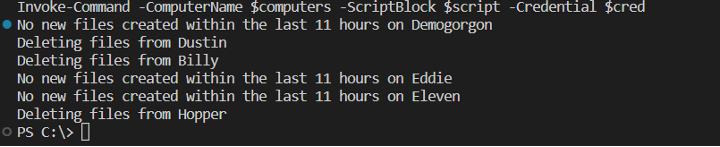
It is funny — I spent all of this time creating a fake scenario to showcase Test-Path, and to build the scenario, Test-Path was needed to make sure I had a C:\Temp on every machine. My script to make this happen looked like this.
If(!(test-path C:\temp)){
New-Item c:\temp -ItemType Directory -Force
}
New-Item C:\temp\JordansSocial.txt
All of that work to generate a scenario, and valid examples just fall from the sky. Anyway, crisis averted. I need a drink.